In programming, an array
is a collection of elements or items. Arrays store data as elements and retrieve them back when you need them. And if we have a look in JavaScript, Array
is a built-in global object that allows you to store multiple elements at once. In this handbook, I’ll teach you all about arrays in JavaScript. We will try to cover all the array methods.
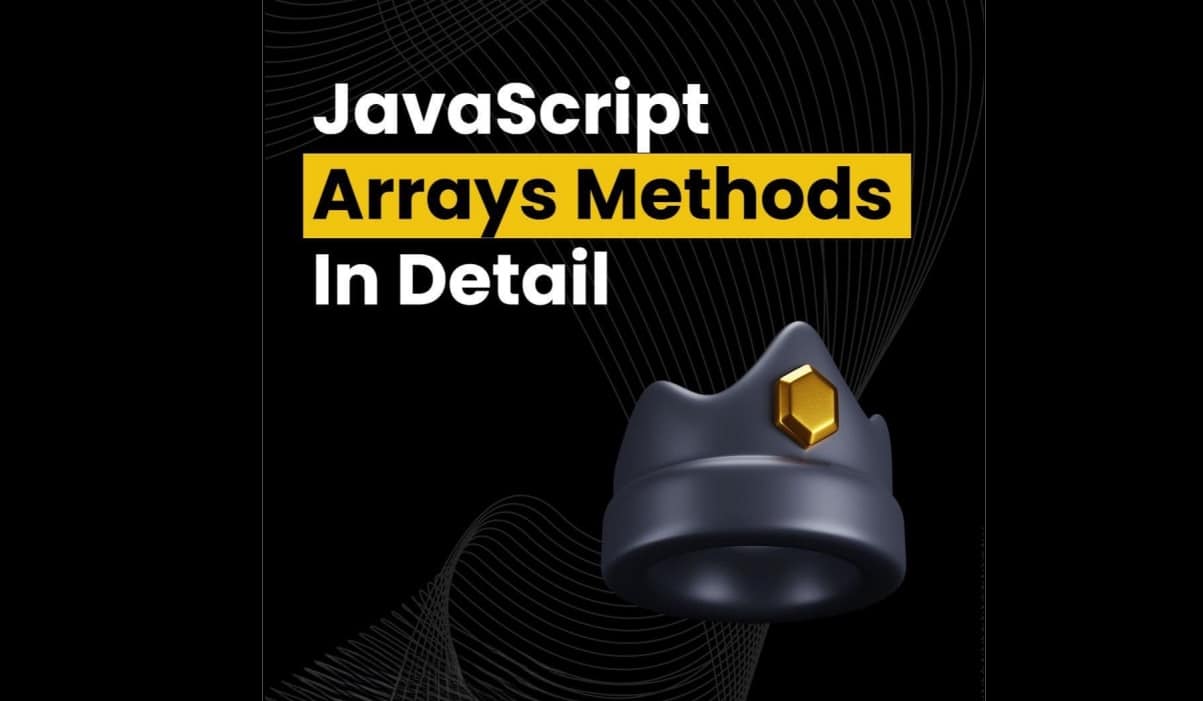