LeetCode patterns for MAANG – Are you tired of solving hundreds of coding problems before interviews? Discover the game-changing solution in our blog – “20 LeetCode Coding Patterns to Follow in MAANG.” Master essential patterns instead of drowning in problems and ace your tech interviews with confidence. Let’s simplify your preparation and unlock your potential!
20 LeetCode Patterns to Follow
Before deep diving into these patterns let me suggest you one thing that Please don’t just LeetCode: follow these patterns instead.
1.Sliding Window
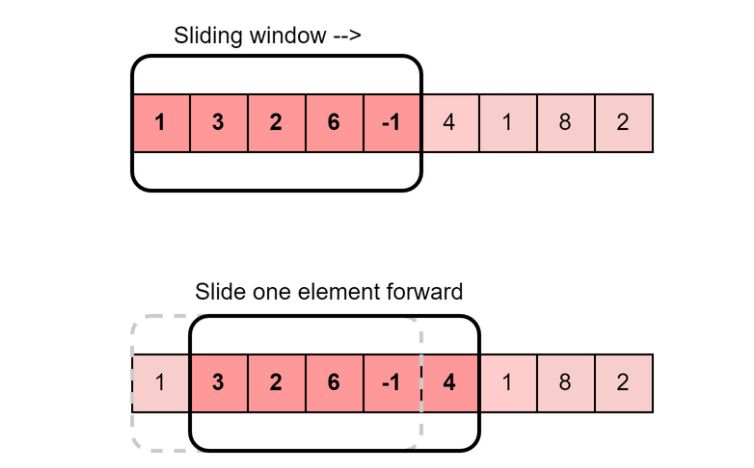
The Sliding window is a problem-solving technique of data structure and algorithm for problems that apply arrays or lists. These problems are painless to solve using a brute force approach in O(n²) or O(n³). However, the Sliding window technique can reduce the time complexity to O(n).
Usage: This algorithmic technique is used when we need to handle the input data in a specific window size.
DS Involved: Array, String, HashTable
Sample Problems:
- Longest Substring with ‘K’ Distinct Characters
- Fruits into Baskets
2. Islands (Matrix Traversal)

The Island pattern describes an efficient way to traverse a matrix. This pattern helps us understand matrix traversal using Depth First Search (DFS) and Breadth First Search (BFS) approaches and their variants.
Usage: This pattern describes all the efficient ways of traversing a matrix (or 2D array).
DS Involved: Matrix, Queue
Sample Problems
- Number of Islands
- Flood Fill
- Cycle in a Matrix
3. Two Pointers – LeetCode patterns for MAANG
Two pointers is really an easy and effective technique that is typically used for searching pairs in a sorted array.
Usage: This technique uses two pointers to iterate input data. Generally, both pointers move in the opposite direction at a constant interval.
DS Involved: Array, String, LinkedList
Sample Problems:
- Squaring a Sorted Array
- Dutch National Flag Problem
- Minimum Window Sort
4. Fast & Slow Pointers

The fast and slow pointer technique (also known as the tortoise and hare algorithm) uses two pointers to determine traits about directional data structures. This can be an array, singly-linked list, or a graph.
Usage: Also known as Hare & Tortoise algorithm. This technique uses two pointers that traverse the input data at different speeds.
DS Involved: Array, String, LinkedList
Sample Problems:
- Middle of the LinkedList
- Happy Number
- Cycle in a Circular Array
5. Merge Intervals – LeetCode patterns for MAANG

A simple approach is to start from the first interval and compare it with all other intervals for overlapping, if it overlaps with any other interval, then remove the other interval from the list and merge the other into the first interval. Repeat the same steps for the remaining intervals after the first. This approach cannot be implemented in better than O(n^2) time.
Usage: This technique is used to deal with overlapping intervals.
DS Involved: Array, Heap
Sample Problems:
- Conflicting Appointments
- Minimum Meeting Rooms
6. Cyclic Sort –
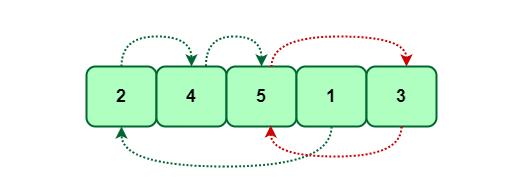
Cycle sort is a comparison sorting algorithm that forces array to be factored into the number of cycles where each of them can be rotated to produce a sorted array. It is theoretically optimal in the sense that it reduces the number of writes to the original array.
Usage: Use this technique to solve array problems where the input data lies within a fixed range.
DS Involved: Array
Sample Problems:
- Find all Missing Numbers
- Find all Duplicate Numbers
- Find the First K Missing Positive Numbers
7. In-place Reversal of a LinkedList
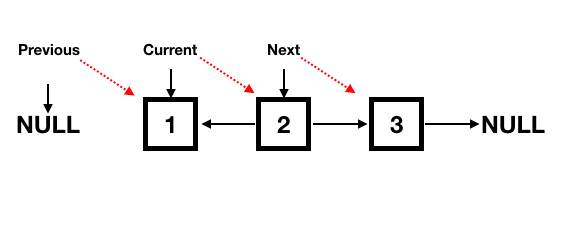
In-place Reversal of a Linked List pattern which is very useful to solve the problems involving reversal of a Linked List with the constraint that we need to do it in-place without using extra memory.
Usage: This technique describes an efficient way to reverse the links between a set of nodes of a LinkedList. Often, the constraint is that we need to do this in-place, i.e., using the existing node objects and without using extra memory.
DS Involved: LinkedList
Sample Problems:
- Reverse every K-element Sub-list
- Rotate a LinkedList
8. Breadth-First Search – LeetCode patterns for MAANG

Breadth First Search (BFS) algorithm traverses a graph in a breadthward motion and uses a queue to remember to get the next vertex to start a search, when a dead end occurs in any iteration.
Usage: This technique is used to solve problems involving traversing trees or graphs in a breadth-first search manner
DS Involved: Tree, Graph, Matrix, Queue
Sample Problems:
- Binary Tree Level Order Traversal
- Minimum Depth of a Binary Tree
- Connect Level Order Siblings
9. Depth First Search
Depth first Search or Depth first traversal is a recursive algorithm for searching all the vertices of a graph or tree data structure. Traversal means visiting all the nodes of a graph.
Usage: This technique is used to solve problems involving traversing trees or graphs in a depth-first search manner.
DS Involved: Tree, Graph, Matrix
Sample Problems:
- Path With Given Sequence
- Count Paths for a Sum
10. Two Heaps
As the name suggests, the two heaps pattern uses either two min-heaps, two max-heaps, or a min-heap and a max-heap simultaneously to solve the problem.
Usage: In many problems, we are given a set of elements that can be divided into two parts. We are interested in knowing the smallest element in one part and the biggest element in the other part. As the name suggests, this technique uses a Min-Heap to find the smallest element and a Max-Heap to find the biggest element.
DS Involved: Heap, Array
Sample Problems:
- Find the Median of a Number Stream
- Next Interval
11. Subsets
A subset is nothing but any possible combination of the original array (or a set). We can have 2^(size of the array) i.e. 2^n subsets of an array.
Usage: Use this technique when the problem asks to deal with permutations or combinations of a set of elements.
DS Involved: Queue, Array, String
Sample Problems:
- String Permutations by changing case
- Unique Generalized Abbreviations
12. Modified Binary Search
The modified binary search pattern is an extension of the traditional binary search algorithm and can be applied to a wide range of problems. Implementing a modified binary search algorithm involves making specific changes to the standard binary search logic based on the requirements.
Usage: Use this technique to search a sorted set of elements efficiently.
DS Involved: Array
Sample Problems:
- Ceiling of a Number
- Bitonic Array Maximum
13. Bitwise XOR
Bitwise XOR operator is also known as Exclusive OR. In the bitwise exclusive OR operator (XOR), two operands are required, and these two operands are separated by the XOR symbol, i.e., ‘^’.
Usage: This technique uses the XOR operator to manipulate bits to solve problems.
DS Involved: Array, Bits
Sample Problems:
- Two Single Numbers
- Flip and Invert an Image
14. Top ‘K’ Elements
The top K elements pattern is a technique that aims to return a given number of the most frequent/largest/smallest elements in a given set. The key data structure of solving the top K elements problems is heap.
Usage: This technique is used to find top/smallest/frequently occurring ‘K’ elements in a set.
DS Involved: Array, Heap, Queue
Sample Problems:
- ‘K’ Closest Points to the Origin
- Maximum Distinct Elements
15. K-way Merge
K-way merge algorithms or multiway merges are a specific type of sequence merge algorithms that specialize in taking in k sorted lists and merging them into a single sorted list. These merge algorithms generally refer to merge algorithms that take in a number of sorted lists greater than two.
Usage: This technique helps us solve problems that involve a list of sorted arrays.
DS Involved: Array, Queue, Heap
Sample Problems:
- Kth Smallest Number in M Sorted Lists
- Kth Smallest Number in a Sorted Matrix
16. Topological Sort
The topological sort algorithm takes a directed graph and returns an array of the nodes where each node appears before all the nodes it points to. The ordering of the nodes in the array is called a topological ordering.
Usage: Use this technique to find a linear ordering of elements that have dependencies on each other.
DS Involved: Array, HashTable, Queue, Graph
Sample Problems:
- Tasks Scheduling
- Alien Dictionary
17. 0/1 Knapsack
The 0/1 knapsack problem means that the items are either completely or no items are filled in a knapsack. For example, we have two items having weights 2kg and 3kg, respectively.
Usage: This technique is used to solve optimization problems. Use this technique to select elements that give maximum profit from a given set with a limitation on capacity and that each element can only be picked once.
DS Involved: Array, HashTable
Sample Problems:
- Equal Subset Sum Partition
- Minimum Subset Sum Difference
18. Fibonacci Numbers
The Fibonacci Sequence or Series is a set of numbers formed by adding two numbers preceding the following number.
Usage: Use this technique to solve problems that follow the Fibonacci numbers sequence, i.e., every subsequent number is calculated from the last few numbers.
DS Involved: Array, HashTable
Sample Problems:
- Staircase
- House Thief
19. Palindromic Subsequence
A palindromic subsequence in DSA is a non-contiguous sequence of elements within a larger sequence that reads the same forwards and backwards. It may skip elements in between and still maintain the palindrome property.
Usage: This technique is used to solve optimization problems related to palindromic sequences or strings.
DS Involved: Array, HashTable
Sample Problems:
- Longest Palindromic Subsequence
- Minimum Deletions in a String to make it a Palindrome
20. Longest Common Substring
The Longest Common Substring is a problem in computer science and algorithms where the objective is to find the longest string that is a substring of two or more input strings.
Usage: Use this technique to find the optimal part of a string/sequence or set of strings/sequences.
DS Involved: Array, HashTable
Sample Problems:
- Maximum Sum Increasing Subsequence
- Edit Distance
- Between Two Sets